Circle
Circle
Bases: DrawingObject
A class to create circles in the tikz environment.
Parameters:
Name | Type | Description | Default |
---|---|---|---|
center |
Union[Tuple[float, float], Point]
|
Pair of floats representing the center of the circle |
required |
radius |
float
|
Length (in cm) of the radius |
required |
options |
str
|
String containing the drawing options (e.g, "Blue") |
''
|
action |
str
|
The type of TikZ action to use. Default is "draw". |
'draw'
|
center
property
writable
Returns a Point object representing the center of the circle.
Examples
Here we create several circles, making use of the action
parameter.
from tikzpy import TikzPicture
tikz = TikzPicture()
tikz.circle((0, 0), 1.25) #action="draw" by default
tikz.line((0, 0), (0, 1.25), options="dashed")
tikz.circle((3, 0), 1, options="thick, fill=red!60", action="filldraw")
tikz.circle((6, 0), 1.25, options="Green!50", action="fill")
tikz.show()
We can also use circles to create the Hawaiian Earing.
from tikzpy import TikzPicture
tikz = TikzPicture()
radius = 5
for i in range(1, 60):
n = radius / i
tikz.circle((n, 0), n)
tikz.show()
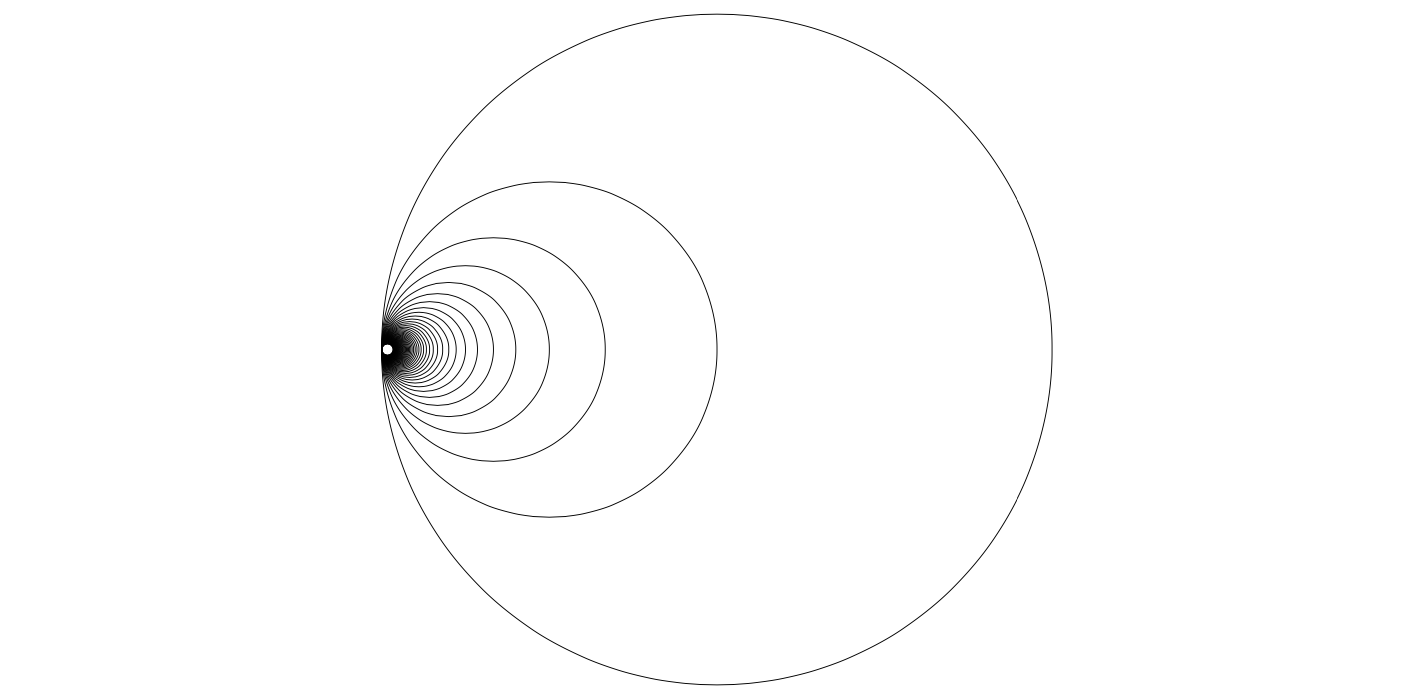